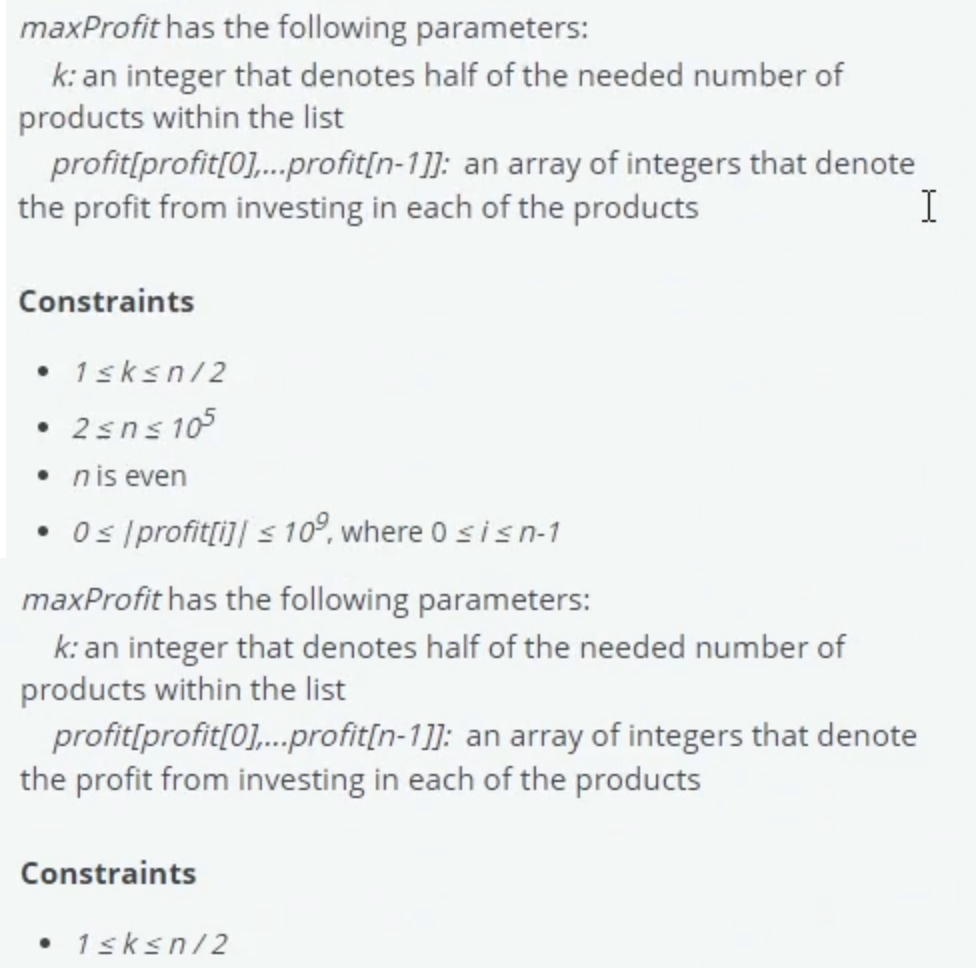
stock picker leetcode
Stock Picker LeetCode: Mastering the Art of Profitable Stock Trading
This in-depth article delves into the fascinating world of stock picking algorithms, specifically exploring how to tackle the "stock picker" LeetCode problems. We'll dissect various approaches, analyze time complexities, and equip you with the knowledge to excel in these challenges. Understanding stock picker LeetCode problems is crucial for anyone looking to dive into algorithm design and coding. This knowledge extends beyond LeetCode and applies to real-world stock trading, and optimization more generally!
Introduction to Stock Picker LeetCode
Stock picker LeetCode problems revolve around identifying optimal buy and sell points in a given stock price sequence to maximize profit. These problems challenge you to strategize effectively to extract the highest possible gains. Your goal is always to purchase at a lower price and sell at a higher price. This task forms a core competency in stock picker LeetCode.
Problem 1: Basic Stock Picker LeetCode – Finding the Maximum Profit
The Task
Given an array of stock prices, find the maximum profit that could be made by buying and selling the stock once. In stock picker LeetCode problems, it is usually assumed only a single transaction can take place. The approach will adapt and enhance for scenarios with multiple possible transactions.
How-To: Using the Single Pass Algorithm for maximum Stock Picker LeetCode Profit
Algorithm:
- Initialize
min_price
to a very high value, andmax_profit
to 0. - Iterate through the price array:
- If the current price is lower than
min_price
, updatemin_price
to the current price. - If the difference between the current price and
min_price
is greater thanmax_profit
, updatemax_profit
.
- If the current price is lower than
def max_profit(prices):
min_price = float('inf')
max_profit = 0
for price in prices:
min_price = min(min_price, price)
max_profit = max(max_profit, price - min_price)
return max_profit
Time and Space Complexity: (O(n), O(1)) for Stock Picker LeetCode
The single pass algorithm provides optimal time complexity to address the challenge in stock picker LeetCode.
Problem 2: Stock Picker LeetCode with Multiple Transactions
Source: leetcode.com
The Task
This extends the previous example to allowing for multiple buy and sell transactions.
Source: medium.com
How-To: Using the Dynamic Programming approach to stock picker LeetCode
Algorithm:
- Create a 2D array
dp
to store maximum profits. (This is dynamic programming at its finest in Stock Picker LeetCode!). dp[i][j]
represents the maximum profit achievable until day i, with j transactions.- Iterate to fill the
dp
table using the recurrence relation.
Stock Picker LeetCode Solution and time-space considerations
This solution demonstrates effective use of dynamic programming and results in efficient solving techniques of Stock Picker LeetCode problems. (Space complexity is potentially impacted if very high numbers of transactions and high day-count arrays are involved)
#Example of dynamic programming usage for multiple stock transactions -Stock Picker LeetCode
Source: ytimg.com
Problem 3: Dealing with Transaction Costs
The Task
Consider the scenarios with transaction costs in stock picker LeetCode! How does this modify the previous solutions?
How-To: Incorporating Transaction Fees into the stock picker LeetCode algorithm
Source: algo.monster
To include transaction costs you adapt the base algorithmic framework and track not only the profit gain/loss but the current asset state in the dynamic approach to stock picker LeetCode problem solving. These concepts can generalize easily to stock picker LeetCode optimization in finance contexts more broadly!
Handling Different Constraints in Stock Picker LeetCode
Stock Picker LeetCode often comes with various constraints, such as time limits, transaction limits, or specific stock behaviour types. The most effective solution method hinges on carefully analyzing those requirements and then deploying appropriate algorithm engineering in stock picker LeetCode, based on whether to use greedy, dynamic, divide and conquer, etc techniques.
Source: gitbooks.io
Stock Picker LeetCode Edge Cases
Recognizing and mitigating for edge cases is crucial. This often comes in the form of empty data arrays.
Advanced Strategies for Stock Picker LeetCode
Beyond basic algorithms in Stock Picker LeetCode, the problem area expands further and involves advanced tools and techniques. This can vary depending on what specific details on Stock Picker LeetCode you are trying to address (e.g. forecasting in financial math).
Common Errors and Troubleshooting in Stock Picker LeetCode
Beware of potential pitfalls such as off-by-one errors, incorrect handling of initial conditions (e.g. with transactions), improper data handling strategies in Stock Picker LeetCode solutions. Proper and concise debugging techniques matter as they relate directly to effective code.
Conclusion on Stock Picker LeetCode
This guide offers an excellent approach and strategies to understanding stock picker LeetCode, including dynamic programming and single-pass solutions. Recognizing problem variants (number of transactions, constraints) enables development of the appropriate algorithm solutions and further fine-tunes problem-solving ability using Stock Picker LeetCode practice. Efficient stock picking for real-world applications leverages understanding the foundational stock picker LeetCode fundamentals!
Future Directions
We explore future developments in algorithms as the financial landscape evolves and these problems advance! This stock picker LeetCode exploration demonstrates the crucial application of advanced computing principles across numerous application domains.