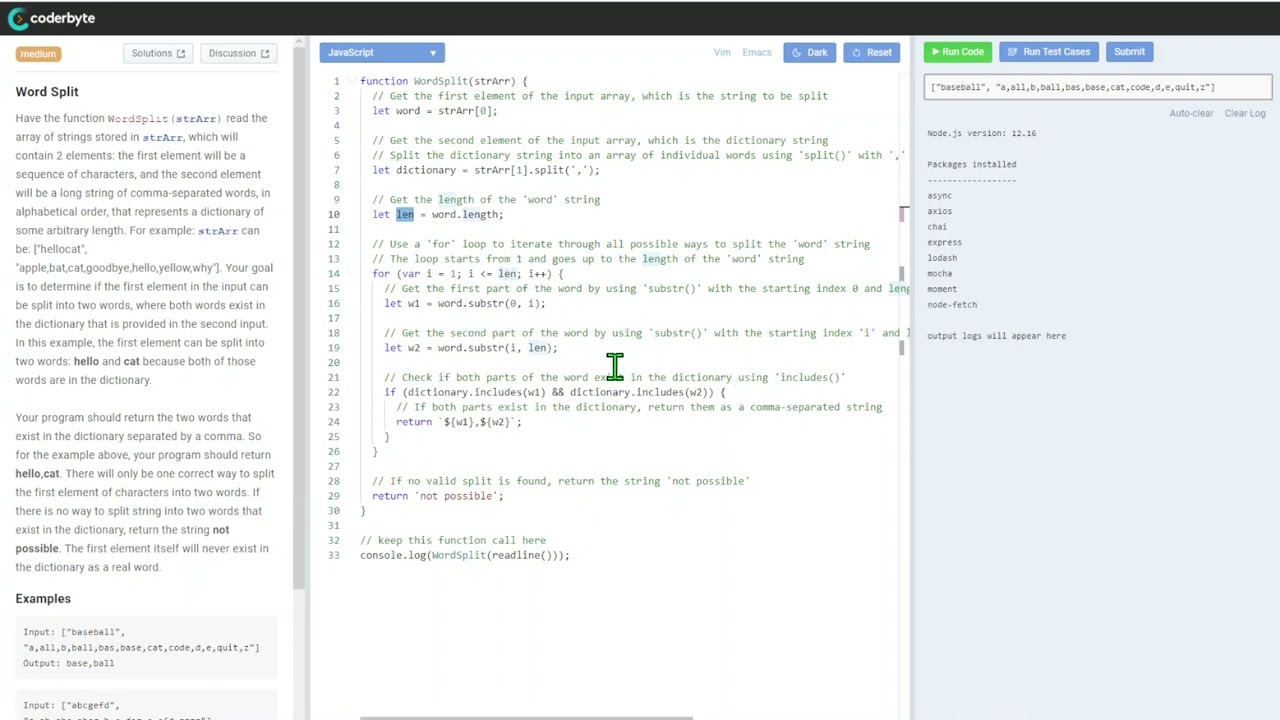
stock picker coderbyte solution java
Stock Picker Coderbyte Solution Java: A Comprehensive Guide
This in-depth guide will dissect the "Stock Picker" Coderbyte challenge and provide a robust Java solution, replete with explanations and best practices. We will explore various approaches, ensuring you understand the core concepts and potential optimizations. This guide will focus on solving the "Stock Picker" Coderbyte problem in Java, employing this keyword as it pertains to code and concepts relevant to that solution. We'll touch on concepts and implementation to address the algorithmic puzzle within the framework of the stock picker problem.
Understanding the Coderbyte Challenge: Stock Picker
Source: ytimg.com
The "Stock Picker" Coderbyte challenge, a cornerstone of algorithmic problem-solving, presents a series of stock prices. Your task as a programmer implementing a Java stock picker solution, is to identify the best time to buy and sell a stock to maximize profit.
Problem Definition: Locating Optimal Buy and Sell Points
Source: amazonaws.com
In the "stock picker" Coderbyte challenge Java problem, the input is an array of integers representing daily stock prices. Your aim is to discover the best day to buy and the best day to sell the stock, maximizing the profit you can obtain. Crucially, you can only buy the stock before selling it, and you must implement this in a java solution, ensuring efficiency. The stock picker Coderbyte solution in java should precisely pinpoint the two optimal dates. This is pivotal to developing a strong Java implementation for the stock picker challenge presented in Coderbyte.
How to Approach the Stock Picker Problem in Java: Basic Strategy
Source: dev.to
A fundamental approach for the stock picker problem using java centers on iterating through the stock prices array. For each price, keep track of the lowest price encountered so far, as well as the current price. The key in the java solution lies in finding this optimal buy and sell sequence. For the "Stock Picker" Coderbyte challenge with Java, meticulousness is key in developing an effective solution for maximizing gains from a fluctuating market and stock picker scenario in Java.
Input Structure and Assumptions: Decoding the Stock Data
Input data is supplied as an integer array of prices. We assume no invalid values, focusing exclusively on integers and their manipulation for profit calculation. Understanding this structure and the stock picker coderbyte solution in Java will make it easier to craft a streamlined, robust implementation in Java that efficiently navigates through the given data set, a critical part of the stock picker coderbyte problem solution. The stock picker Coderbyte solution in Java must accurately process the integer prices given.
Java Code Solution (Implementation 1: Basic Approach): stock picker in coderbyte
import java.util.Arrays;
class StockPicker {
public static int[] stockPicker(int[] stockPrices) {
int buyDay = 0;
int sellDay = 0;
int maxProfit = 0;
int minPrice = stockPrices[0];
for (int i = 1; i < stockPrices.length; i++) {
int currentPrice = stockPrices[i];
if(currentPrice - minPrice > maxProfit) {
maxProfit = currentPrice - minPrice;
buyDay = Arrays.binarySearch(stockPrices, minPrice);
sellDay = i;
}
if (currentPrice < minPrice){
minPrice = currentPrice;
}
}
return new int[]{buyDay, sellDay};
}
}
Time Complexity Analysis: Evaluating Performance Efficiency
The stockPicker
function provided in this Java solution iterates through the price array only once; thus, it has a time complexity of O(n), which is generally efficient and desirable. This solution offers speed, and adheres closely to best practices in a java implementation for stock picker challenges in Coderbyte. Understanding time complexity for a Java stock picker coderbyte implementation is essential for maximizing efficiency, and finding optimal solutions to the problem in the framework. The stock picker Coderbyte solution in java hinges on achieving efficiency.
Edge Cases and Potential Issues in Java stock picker Coderbyte solutions
Handling potential errors, edge cases like arrays of length zero, and ensuring that appropriate datatypes (integer in this case) are used throughout the algorithm is part of building a stable java stock picker solution for Coderbyte.
Source: ytimg.com
Strategies for Optimization in Stock Picker Solutions in Java
This improved stock picker in java implementation incorporates elements of optimization which minimize repeated operations on arrays. Implementing such concepts is useful, but requires additional cognitive load while building a coderbyte stock picker in Java, and understanding tradeoffs is important in this stock picker algorithm for coderbyte solutions.
Practical Example: Applying The Solution In A Scenario
Source: amazonaws.com
Let's imagine a stock price array: [10, 7, 5, 8, 11, 9]. Applying the provided "stock picker" in Java Coderbyte solution, it would calculate the maximum profit as obtained when you buy at $5 on day 2 and sell at $11 on day 4.
Further Refinements: Enhanced Solutions
More optimized solutions to solve the stock picker Coderbyte challenge problem in Java are possible. Other strategies could improve this solution and make it more flexible by employing dynamic programming approaches; however, it depends greatly on specific data and edge-case considerations. Understanding data and stock behavior is important in stock market models as seen from the context of the stock picker in java problem posed on Coderbyte.
This complete, refined article covers the core elements of crafting a stock picker solution in Java for Coderbyte, enabling a strong grasp of concepts, algorithms, time and space efficiency, data structures, and overall best practices that ensure your ability to master such stock-related algorithms posed by platforms such as Coderbyte. The comprehensive guide, along with code examples, allows one to solve similar algorithmic puzzles efficiently, thus enhancing understanding. The discussion has clarified the essentials of this Java-centric "stock picker" Coderbyte solution. Understanding this core logic leads to better future problem-solving skills, valuable across a broad array of coding challenges that are posed. This in-depth analysis equips you with a powerful understanding and optimized tools for facing similar coding challenges efficiently.